Tabs
Organizes content into conditionally rendered sections, controlled by a group of triggers.
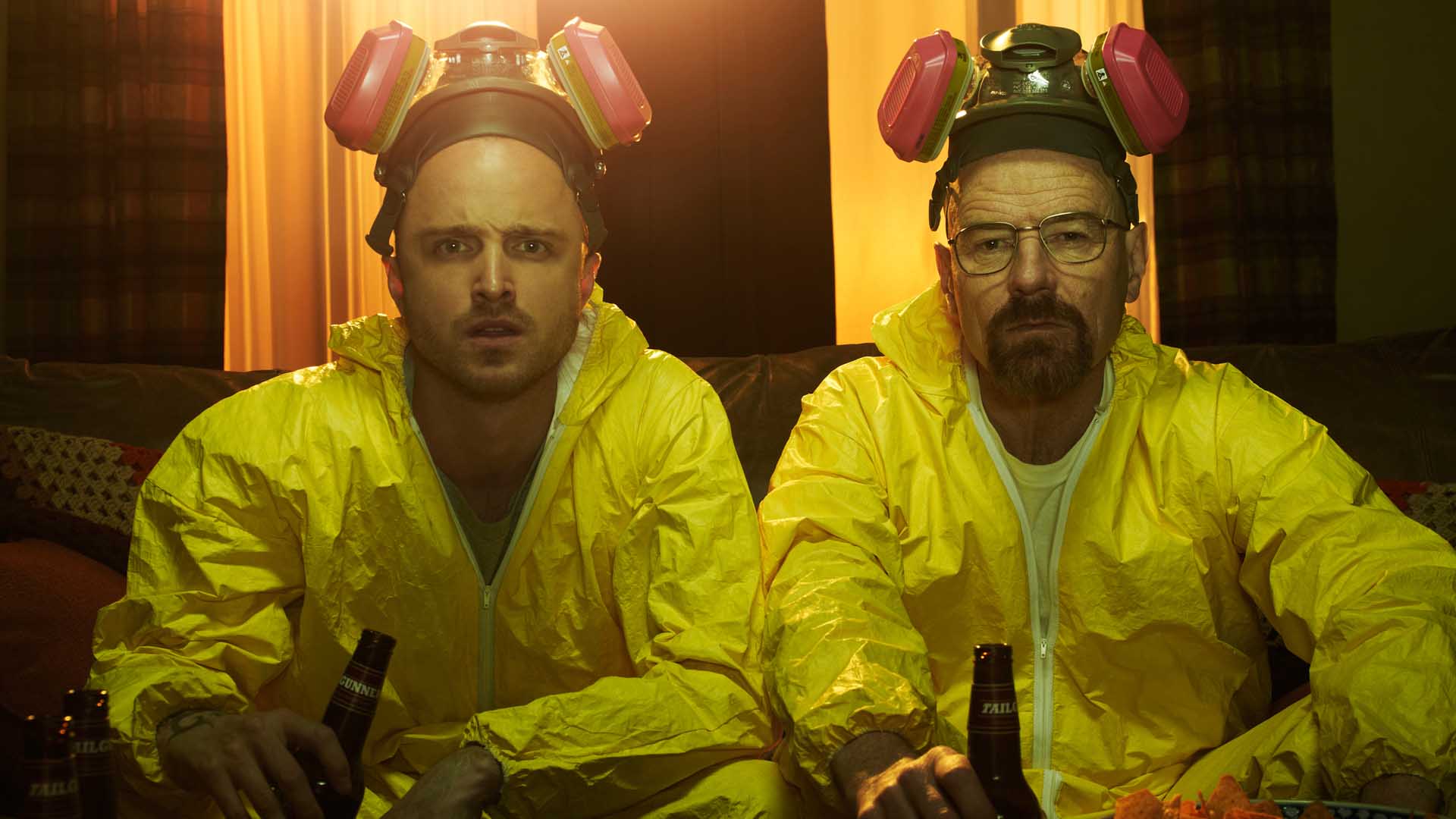
Breaking Bad
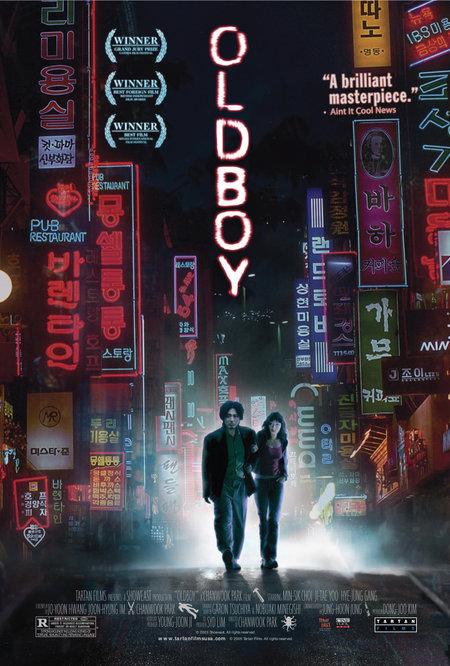
Oldboy
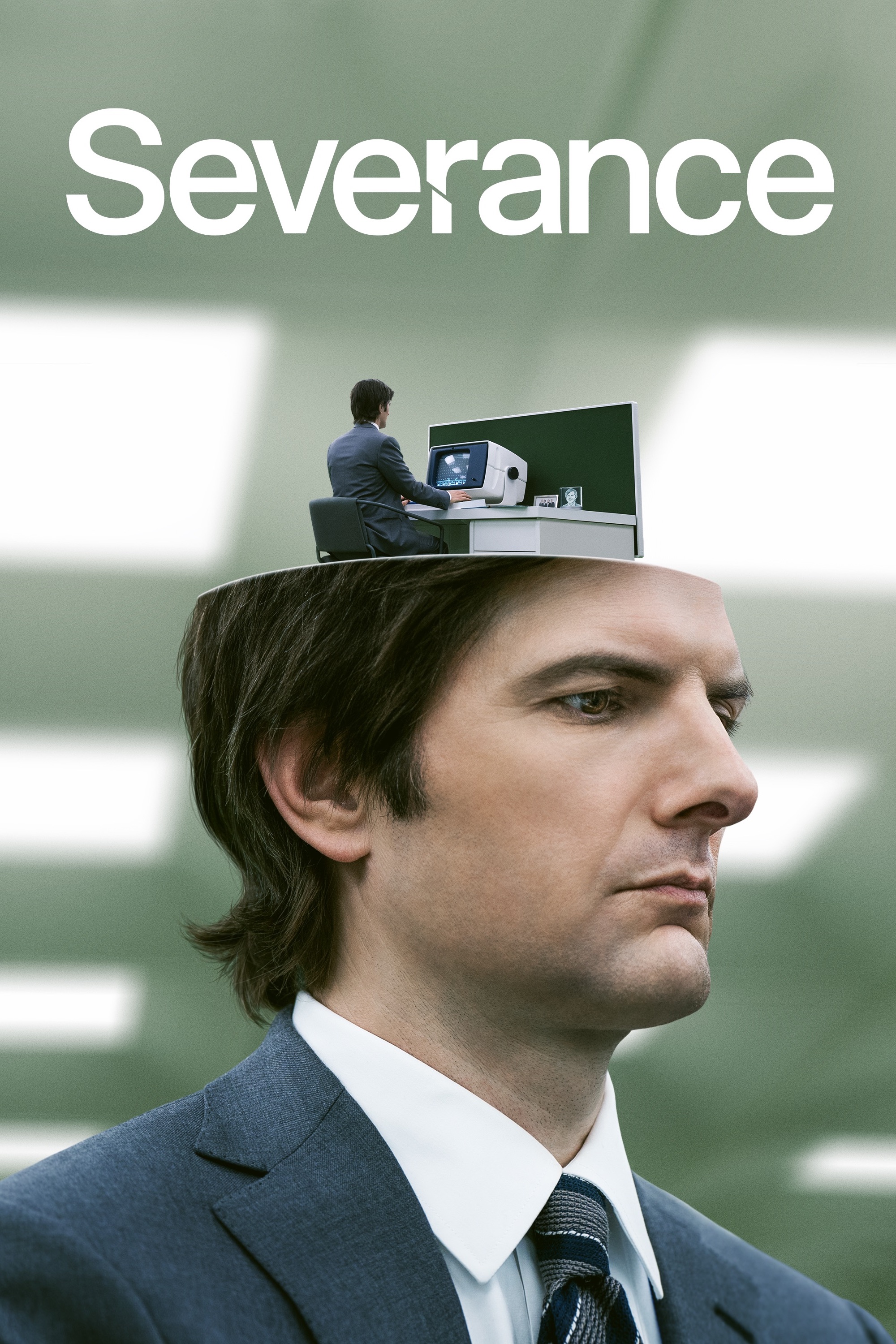
Severance
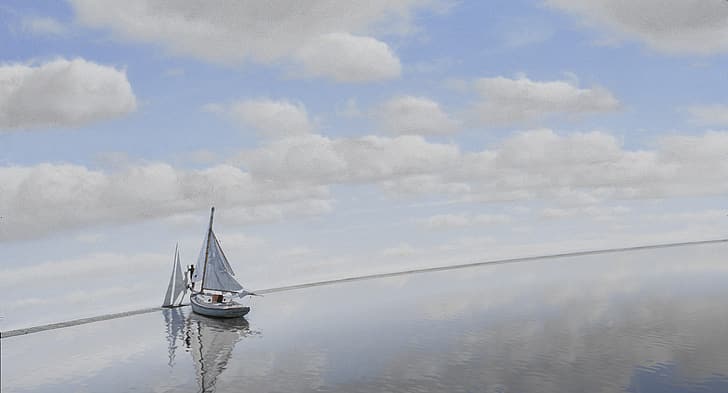
The Truman Show
Props
Features
- 🎹 Keyboard navigation
- 🧠 Smart focus management
- 🔄 Horizontal and vertical orientation
🌐 RTL support(incoming!)
Usage
<script lang="ts"> import { Tabs } from "melt/builders";
const tabs = new Tabs() const tabIds = ["Tab 1", "Tab 2", "Tab 3"];</script>
<div {...tabs.triggerList}> {#each tabIds as id} <button {...tabs.getTrigger(id)}> {id} </button> {/each}</div>
{#each tabIds as id} <div {...tabs.getContent(id)}> {id} </div>{/each}
<script lang="ts"> import { Tabs } from "melt/components";
const tabIds = ["Tab 1", "Tab 2", "Tab 3"];</script>
<Tabs> {#snippet children(tabs)} <div {...tabs.triggerList}> {#each tabIds as id} <button {...tabs.getTrigger(id)}> {id} </button> {/each} </div>
{#each tabIds as id} <div {...tabs.getContent(id)}> {id} </div> {/each} {/snippet}</Tabs>
API Reference
Constructor Props
The props that are passed when calling
new Tabs()
new Tabs()
export type TabsProps<T extends string = string> = { /** * If `true`, the value will be changed whenever a trigger is focused. * * @default true */ selectWhenFocused?: MaybeGetter<boolean | undefined>; /** * If the the trigger selection should loop when navigating with the arrow keys. * * @default true */ loop?: MaybeGetter<boolean | undefined>; /** * The orientation of the tabs. * * @default "horizontal" */ orientation?: MaybeGetter<"horizontal" | "vertical" | undefined>; /** * The default value for `tabs.value` * * When passing a getter, it will be used as source of truth, * meaning that `tabs.value` only changes when the getter returns a new value. * */ value: MaybeGetter<T>; /** * Called when the `Tabs` instance tries to change the active tab. */ onValueChange?: (active: T) => void;};
export type TabsProps<T extends string = string> = { /** * If `true`, the value will be changed whenever a trigger is focused. * * @default true */ selectWhenFocused?: MaybeGetter<boolean | undefined>; /** * If the the trigger selection should loop when navigating with the arrow keys. * * @default true */ loop?: MaybeGetter<boolean | undefined>; /** * The orientation of the tabs. * * @default "horizontal" */ orientation?: MaybeGetter<"horizontal" | "vertical" | undefined>; /** * The default value for `tabs.value` * * When passing a getter, it will be used as source of truth, * meaning that `tabs.value` only changes when the getter returns a new value. * */ value: MaybeGetter<T>; /** * Called when the `Tabs` instance tries to change the active tab. */ onValueChange?: (active: T) => void;};
Methods
The methods returned from
new Tabs()
new Tabs()
-
getTrigger
(value: T) => {readonly "data-melt-tabs-trigger": Treadonly "data-active": "" | undefinedreadonly tabindex: 0 | -1readonly role: "tab"readonly "aria-selected": booleanreadonly "aria-controls": stringreadonly "data-orientation": "horizontal" | "vertical"readonly onclick: () => Treadonly onkeydown: (e: KeyboardEvent) => voidreadonly id: string}(value: T) => {readonly "data-melt-tabs-trigger": Treadonly "data-active": "" | undefinedreadonly tabindex: 0 | -1readonly role: "tab"readonly "aria-selected": booleanreadonly "aria-controls": stringreadonly "data-orientation": "horizontal" | "vertical"readonly onclick: () => Treadonly onkeydown: (e: KeyboardEvent) => voidreadonly id: string}Gets the attributes and listeners for a tab trigger. Requires an identifying tab value. -
getContent
(value: T) => {readonly "data-melt-tabs-content": ""readonly hidden: booleanreadonly "data-active": "" | undefinedreadonly role: "tabpanel"readonly id: stringreadonly "aria-labelledby": stringreadonly "data-orientation": "horizontal" | "vertical"}(value: T) => {readonly "data-melt-tabs-content": ""readonly hidden: booleanreadonly "data-active": "" | undefinedreadonly role: "tabpanel"readonly id: stringreadonly "aria-labelledby": stringreadonly "data-orientation": "horizontal" | "vertical"}Gets the attributes and listeners for the tabs contents. Requires an identifying tab value.
Properties
The properties returned from
new Tabs()
new Tabs()
-
selectWhenFocused
booleanboolean -
loop
booleanboolean -
orientation
"horizontal" | "vertical""horizontal" | "vertical" -
value
TTThe current selected tab. -
triggerList
{readonly "data-melt-tabs-trigger-list": ""readonly role: "tablist"readonly "aria-orientation": "horizontal" | "vertical"readonly "data-orientation": "horizontal" | "vertical"}{readonly "data-melt-tabs-trigger-list": ""readonly role: "tablist"readonly "aria-orientation": "horizontal" | "vertical"readonly "data-orientation": "horizontal" | "vertical"}The attributes for the list that contains the tab triggers.